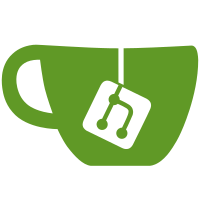
The DisplayApp class isn't used in the Screen base class and most screens, so requiring it is pointless. In this commit, DisplayApp pointers were added to screens which use it and the explicit Screen constructor was removed in those screens.
62 lines
2.1 KiB
C++
62 lines
2.1 KiB
C++
#pragma once
|
|
|
|
#include <array>
|
|
#include <memory>
|
|
|
|
#include "displayapp/screens/Screen.h"
|
|
#include "displayapp/screens/ScreenList.h"
|
|
#include "components/datetime/DateTimeController.h"
|
|
#include "components/settings/Settings.h"
|
|
#include "components/battery/BatteryController.h"
|
|
#include "displayapp/screens/Symbols.h"
|
|
#include "displayapp/screens/Tile.h"
|
|
|
|
namespace Pinetime {
|
|
namespace Applications {
|
|
namespace Screens {
|
|
class ApplicationList : public Screen {
|
|
public:
|
|
explicit ApplicationList(DisplayApp* app,
|
|
Pinetime::Controllers::Settings& settingsController,
|
|
const Pinetime::Controllers::Battery& batteryController,
|
|
const Pinetime::Controllers::Ble& bleController,
|
|
Controllers::DateTime& dateTimeController);
|
|
~ApplicationList() override;
|
|
bool OnTouchEvent(TouchEvents event) override;
|
|
|
|
private:
|
|
DisplayApp* app;
|
|
auto CreateScreenList() const;
|
|
std::unique_ptr<Screen> CreateScreen(unsigned int screenNum) const;
|
|
|
|
Controllers::Settings& settingsController;
|
|
const Pinetime::Controllers::Battery& batteryController;
|
|
const Pinetime::Controllers::Ble& bleController;
|
|
Controllers::DateTime& dateTimeController;
|
|
|
|
static constexpr int appsPerScreen = 6;
|
|
|
|
// Increment this when more space is needed
|
|
static constexpr int nScreens = 2;
|
|
|
|
static constexpr std::array<Tile::Applications, appsPerScreen * nScreens> applications {{
|
|
{Symbols::stopWatch, Apps::StopWatch},
|
|
{Symbols::clock, Apps::Alarm},
|
|
{Symbols::hourGlass, Apps::Timer},
|
|
{Symbols::shoe, Apps::Steps},
|
|
{Symbols::heartBeat, Apps::HeartRate},
|
|
{Symbols::music, Apps::Music},
|
|
|
|
{Symbols::paintbrush, Apps::Paint},
|
|
{Symbols::paddle, Apps::Paddle},
|
|
{"2", Apps::Twos},
|
|
{Symbols::chartLine, Apps::Motion},
|
|
{Symbols::drum, Apps::Metronome},
|
|
{Symbols::map, Apps::Navigation},
|
|
}};
|
|
ScreenList<nScreens> screens;
|
|
};
|
|
}
|
|
}
|
|
}
|